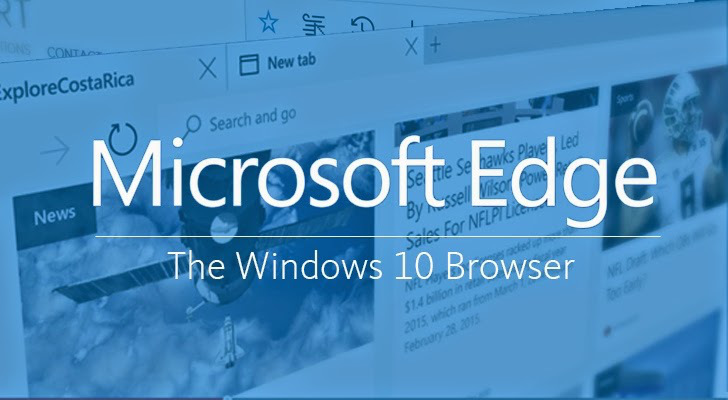
[Java – Webdriver 06] Run automation trên Windows 10 – Microsoft Edge
Nhân dịp Microsoft tung ra bản Windows 10, sau khi upgrade từ bản Windows 8.1 thành công, mình dùng hơn 2 tháng thì chưa thấy issue gì trong quá trình làm việc (automation – manual), chốt lại 1 câu là chạy khá mướt.
Ngày 23/07/2015, Microsoft thông báo chính thức về việc tự động hóa cho trình duyệt Microsft Edge thông qua Selenium Webdriver (link), có hiệu lực từ phiên bản Selenium 2.47 (link). Trình duyệt Edge được đặt là trình duyệt mặc định của Windows 10 thay thế hoàn toàn cho Internet Explorer kể từ bây giờ.
Có một số hạn chế trên Edge:
- Chưa hỗ trợ run parallel
- Chưa xử lí được alert
- Chưa hỗ trợ mouse hover
- Chỉ hỗ trợ các locator như: ID, CSS, cssSelector, Name, tagName || chưa hỗ trợ: linkText, partialLinkText, xpath
Để chạy được trình duyệt Edge cần phải cài đặt Microsoft Driver (link), tương tự như Chrome/IE driver để khởi tạo/ start trình duyệt. Tải về và cài đặt như các phần mềm thông dụng.
Source demo:
Mình sẽ demo thử 1 vài testcase xem nó chạy như thế nào, review tổng quan để sau này khách hàng có yêu cầu thì phản hồi ngay và đáp ứng được.
- Test01_LoginWithEmptyEmailPassword()
- Test02_LoginWithInvalidEmail()
- Test03_LoginWithIncorectPassword()
- Test04_LoginWithCorrectEmailPassword()
- Test05_SearchAnItemSuccessfully()
package seleniumWebDriver;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.edge.EdgeDriver;
import org.testng.Assert;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;public class WebDriver06_RunOnMicrosoftEdge {
WebDriver driver;@BeforeClass
public void setUp() {
System.setProperty(“webdriver.edge.driver”,
“C:\\Program Files (x86)\\Microsoft Web Driver\\MicrosoftWebDriver.exe”);
driver = new EdgeDriver();
}@Test
public void Test01_LoginWithEmptyEmailPassword() throws Exception {
driver.get(“http://live.guru99.com/index.php/customer/account/login/”);
Thread.sleep(8000);//Click Login button
driver.findElement(By.id(“send2”)).click();
Thread.sleep(2000);//Get error message at ‘Email Address’ field
String errorEmail = driver.findElement(By.id(“advice-required-entry-email”)).getText();//Verify error message display correctly
Assert.assertEquals(errorEmail, “This is a required field.”);//Get error message at ‘Password’ field
String errorPassword = driver.findElement(By.id(“advice-required-entry-pass”)).getText();//Verify error message display correctly
Assert.assertEquals(errorPassword, “This is a required field.”);
}@Test
public void Test02_LoginWithInvalidEmail() throws Exception{
driver.get(“http://live.guru99.com/index.php/customer/account/login/”);
Thread.sleep(8000);//Input correct Username
driver.findElement(By.id(“email”)).sendKeys(“123434234@12312.123123”);//Click Login button
driver.findElement(By.id(“send2”)).click();
Thread.sleep(5000);//Get error message is displayed
String errorMessage = driver.findElement(By.id(“advice-validate-email-email”)).getText();//Verify error message display correctly
Assert.assertEquals(errorMessage, “Please enter a valid email address. For example johndoe@domain.com.”);
}@Test
public void Test03_LoginWithIncorectPassword() throws Exception{
driver.get(“http://live.guru99.com/index.php/customer/account/login/”);
Thread.sleep(8000);//Input correct Username
driver.findElement(By.id(“email”)).sendKeys(“automation@gmail.com”);//Input correct Password
driver.findElement(By.id(“pass”)).sendKeys(“123”);//Click Login button
driver.findElement(By.id(“send2”)).click();
Thread.sleep(3000);//Get error message at ‘Password’ field
String errorPassword = driver.findElement(By.id(“advice-validate-password-pass”)).getText();//Verify message display correctly
Assert.assertEquals(errorPassword, “Please enter 6 or more characters. Leading or trailing spaces will be ignored.”);
}@Test
public void Test04_LoginWithCorrectEmailPassword() throws Exception{
driver.get(“http://live.guru99.com/index.php/customer/account/login/”);
Thread.sleep(5000);//Input correct Username
driver.findElement(By.id(“email”)).sendKeys(“automation@gmail.com”);//Input correct Password
driver.findElement(By.id(“pass”)).sendKeys(“123123”);//Click Login button
driver.findElement(By.id(“send2”)).click();
Thread.sleep(5000);//Verify that ‘Search’ textbox display correctly
driver.findElement(By.id(“search”)).isDisplayed();
}@Test
public void Test05_SearchAnItemSuccessfully() throws Exception{
driver.get(“http://live.guru99.com/index.php/customer/account/login/”);
Thread.sleep(5000);//Input data and click Search
driver.findElement(By.id(“search”)).sendKeys(“SAMSUNG GALAXY”);
driver.findElement(By.id(“search”)).sendKeys(Keys.ENTER);
Thread.sleep(5000);//Verify that ‘Samsung Galaxy’ item display correctly
driver.findElement(By.id(“product-collection-image-3”)).isDisplayed();
}@AfterClass
public void tearDown() {
driver.quit();
}
}
Video demo: