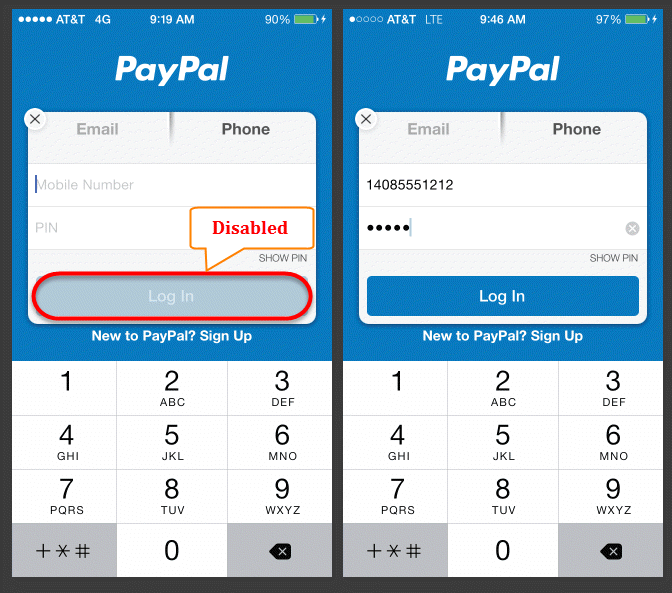
[Java – Webdriver 09] – Kiểm tra phần tử bị disable/ enable
Yêu cầu:
- Phần tử enable: có thể xem, chỉnh sửa giá trị, thực hiện các action như nhập, chọn dữ liệu, kéo, thả,… || phần tử disable: chỉ có thể xem, không được phép chỉnh sửa, không thực hiện được các action như enable
- Kiểm tra phần tử như textbox, checkbox, radiobutton, dropdown, textarea,… đang được enable hay disable. Áp dụng khi check về UI cho 1 page hoặc khách hàng yêu cầu trong 1 page, field nào được phép enable, field nào bị disable
Ví dụ như hình dưới khi check vào checkbox “Contingent Rent” thì 4 textbox “Period End/ Contingent Rent Description/ Days to Report/ Breakover” sẽ được enable => vậy thì trước khi check vào checkbox “Contingent Rent” chúng ta phải xác định 4 textbox “Period End/ Contingent Rent Description/ Days to Report/ Breakover” có phải là đang bị disable hay không, nếu không thì đó là Bug -> Dev code bị miss requirement)
Giải pháp:
- import thư viện: org.openqa.selenium.WebElement;
- Khởi tạo: WebElement locator;
- Kiểm tra hiển thị: locator.isEnabled();
- Xem chi tiết hàm isElementEnabled() trong phần Source demo phía dưới nhé
Site demo: Link
- Như hình dưới, mình sẽ check các element này đang bị disable:
- Password textbox
- Age radio button
- Biography text area
- Job Role 02 dropdown list
- Interest checkbox
- Slider 02 slider
Source demo:
package seleniumWebDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.NoSuchElementException; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.annotations.AfterClass; import org.testng.annotations.BeforeClass; import org.testng.annotations.Test; public class WebDriver09_ElementIsEnabledOnPage { WebDriver driver; @BeforeClass public void setUp() { driver = new FirefoxDriver(); } @Test public void Test01_TextboxIsDisabled() throws Exception { driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); driver.get("http://daominhdam.890m.com"); driver.manage().window().maximize(); String element = "//input[@id='password']"; if (isElementEnabled(driver, element)) { System.out.println("Textbox is enabled"); } else { System.out.println("Textbox is disabled"); } } @Test public void Test02_TextAreaIsDisabled() throws Exception { String element = "//textarea[@id='bio']"; if (isElementEnabled(driver, element)) { System.out.println("Text Area is enabled"); } else { System.out.println("Text Area is disabled"); } } @Test public void Test03_CheckboxIsDisabled() throws Exception { String element = "//*[@id='check-disbaled']"; if (isElementEnabled(driver, element)) { System.out.println("Checkbox is enabled"); } else { System.out.println("Checkbox is disabled"); } } @Test public void Test04_ButtonIsDisabled() throws Exception { String element = "//*[@id='button-disabled']"; if (isElementEnabled(driver, element)) { System.out.println("Button is enabled"); } else { System.out.println("Button is disabled"); } } @Test public void Test05_SliderIsDisabled() throws Exception { String element = "//*[@id='slider-2']"; if (isElementEnabled(driver, element)) { System.out.println("Slider is enabled"); } else { System.out.println("Slider is disabled"); } } @Test public void Test06_RadioButtonIsDisabled() throws Exception { String element = "//input[@id='radio-disabled']"; if (isElementEnabled(driver, element)) { System.out.println("Radio button is enabled"); } else { System.out.println("Radio button is disabled"); } } @Test public void Test07_DropdownListIsDisabled() throws Exception { String element = "//*[@id='job2']"; if (isElementEnabled(driver, element)) { System.out.println("Dropdown List is enabled"); } else { System.out.println("Dropdown List is disabled \n"); } } public boolean isElementEnabled(WebDriver driver, String yourLocator) { try { WebElement locator; locator = driver.findElement(By.xpath(yourLocator)); return locator.isEnabled(); } catch (NoSuchElementException e) { return false; } } @AfterClass public void tearDown() { driver.quit(); } }
Video demo:
https://www.youtube.com/watch?v=zKwOlfmEokI