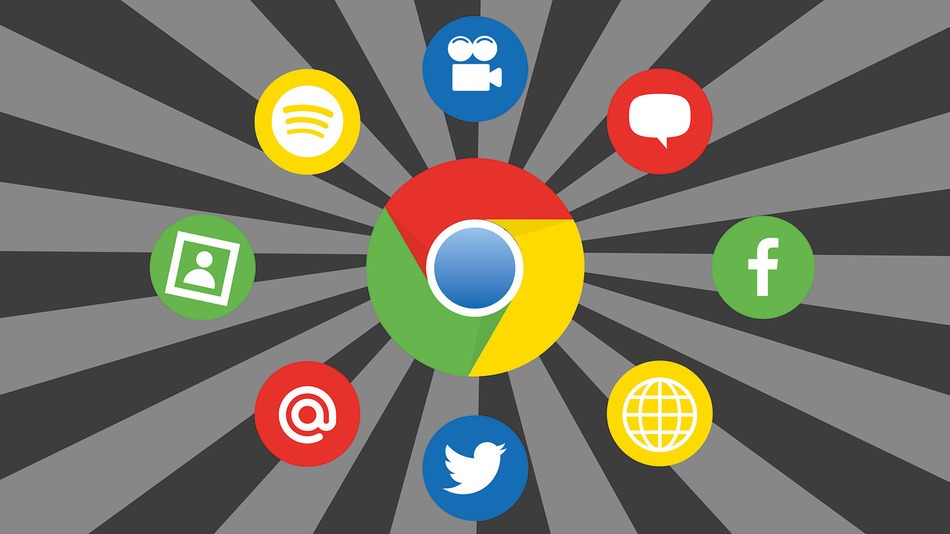
[Java – Webdriver 03] Thêm extensions vào các trình duyệt mặc định
Thông thường khi chúng ta chạy automation, sau khi trình duyệt được khởi tạo thì mặc định các extensions sẽ không được thêm vào. Để làm điều này, chúng ta cần tải về phiên bản offline của extension và add trực tiếp vào trình duyệt lúc khởi tạo.
Ví dụ: Tải về extension là Google translate bản offline cho trình duyệt Chrome
1 – Truy cập vào website Chrome Web Store, search và lấy đường link của extension Google translate
2 – Truy cập vào website Chrome Extension Download, dán đường link của extension Google translate và tải về máy
Firefox:
FirefoxProfile profile = new FirefoxProfile();
//File .xpi là định dạng extesion của trình duyệt Firefox
File extension = new File(“Extension.xpi”);
profile.addExtension(extension);
WebDriver driver = new FirefoxDriver(profile);
Chrome:
System.setProperty(“webdriver.chrome.driver”,”..\\chromedriver.exe”);
//File .crx hoặc .zip là định dạng extesion của trình duyệt Chrome
File file = new File(“Extension.crx”);
ChromeOptions options = new ChromeOptions();
options.addExtensions(file);
WebDriver driver = new ChromeDriver(options);
Safari:
File file = new File(“Extension.safariextz”);
//File .safariextz là định dạng extesion của trình duyệt Safari
SafariOptions options = new SafariOptions();
options.addExtensions(file);
WebDriver driver = new SafariDriver(options);
Opera:
OperaProfile operaProfile = new OperaProfile();
//File .oex là định dạng extesion của trình duyệt Opera
File file = new File(“C:\\Extension.oex”);
operaProfile.addExtension(file);
WebDriver driver = new OperaDriver(operaProfile);
Source demo:
import java.io.File;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.firefox.FirefoxProfile;
import org.openqa.selenium.safari.SafariDriver;
import org.openqa.selenium.safari.SafariOptions;
import org.testng.annotations.Test;
import com.opera.core.systems.OperaDriver;
import com.opera.core.systems.OperaProfile;public class WebDriver02_AddExtensions {
@Test (priority = 1)
public void addExtensionToFirefox() throws Exception {
FirefoxProfile profile = new FirefoxProfile();
//File .xpi là định dạng extesion của trình duyệt Firefox
File extension = new File(“\\11-AutomationTestingDemo\\lib\\firebug-2.0.1.xpi”);
profile.addExtension(extension);
WebDriver driver = new FirefoxDriver(profile);
driver.navigate().to(“http://google.com.vn”);
driver.close();
}@Test (priority = 2)
public void addExtensionToChrome(){
System.setProperty(“webdriver.chrome.driver”,”\\11-AutomationTestingDemo\\lib\\chromedriver.exe”);
//File .crx hoặc .zip là định dạng extesion của trình duyệt Chrome
File file = new File(“\\11-AutomationTestingDemo\\lib\\Google-Translate_v2.0.4.crx”);
ChromeOptions options = new ChromeOptions();
options.addExtensions(file);
WebDriver driver = new ChromeDriver(options);
driver.navigate().to(“http://google.com.vn”);
driver.close();
}@Test (priority = 3)
public void addExtensionToSafari(){
File file = new File(“Extension.safariextz”);
//File .safariextz là định dạng extesion của trình duyệt Safari
SafariOptions options = new SafariOptions();
options.addExtensions(file);
WebDriver driver = new SafariDriver(options);
driver.navigate().to(“http://google.com.vn”);
}@Test (priority = 4)
public void addExtensionToOpera() throws Exception{
OperaProfile operaProfile = new OperaProfile();
//File .oex là định dạng extesion của trình duyệt Opera
File file = new File(“C:\\Extension.oex”);
operaProfile.addExtension(file);
WebDriver driver = new OperaDriver(operaProfile);
driver.navigate().to(“http://google.com.vn”);
}
}
Video demo – Trên máy chỉ có sẵn firefox và chrome nên mình chỉ chạy demo cho 2 trình duyệt này 🙂